Table of Contents
Introduction
How to debug a bash script? you can investigate the causes of the problems so you can apply fixes. Yeah, I will guide you turn on debug bash script. Bash script the essential for DevOps Roles.
Bash scripting is a powerful tool for automating tasks in Linux and Unix environments. However, like any programming language, debugging errors in Bash scripts can be challenging without the right techniques.
In this guide, we’ll explore how to debug Bash scripts effectively, from basic troubleshooting methods to advanced debugging tools. Whether you’re a beginner or an experienced sysadmin, mastering these techniques will save you time and help avoid unnecessary frustrations.
Why Debugging Bash Scripts is Important
Debugging is crucial to ensure your scripts function as intended. Bash scripts often interact with files, processes, and other programs, making it essential to quickly identify and resolve errors. Debugging not only improves script reliability but also deepens your understanding of Bash’s functionality.
How to Debug Bash Scripts: Techniques and Tools
1. Use the set
Command for Debugging
The set
command enables or disables shell options, making it highly useful for debugging. Common options include:
-x
: Displays each command and its arguments as they are executed.-e
: Exits immediately if a command returns a non-zero status.-u
: Treats unset variables as errors.
Example:
#!/bin/bash
set -x # Enable debugging
# Example script
name="Debugging"
echo "Hello, $name!"
set +x # Disable debugging
2. Use bash -x
for Script Debugging
Run your script with the -x
option to trace each command:
bash -x your_script.sh
This provides a detailed execution trace, helping you pinpoint where errors occur.
3. Add echo
Statements for Manual Debugging
Using echo
is a simple yet effective way to debug scripts. Insert echo
statements at key points to check variable values and script flow.
Example:
#!/bin/bash
echo "Starting script..."
value=$((5 + 5))
echo "Value is: $value"
4. Redirect Debug Output to a File
For lengthy scripts, redirect debug output to a file for easier analysis:
bash -x your_script.sh > debug.log 2>&1
5. Use trap
for Error Handling
The trap
command executes a specified action when a signal is received. Use it to clean up resources or log errors.
Example:
#!/bin/bash
trap 'echo "Error occurred at line $LINENO"' ERR
# Example script
cp nonexistent_file.txt /tmp/
6. Advanced Debugging with DEBUG
Hook
The DEBUG
trap executes before each command, allowing for fine-grained debugging.
Example:
#!/bin/bash
trap 'echo "Executing line: $BASH_COMMAND"' DEBUG
echo "This is a test."
value=$((10 + 10))
echo "Value: $value"
Debugging Examples: From Basic to Advanced
Basic Debugging
Scenario: Missing File Error
#!/bin/bash
file="data.txt"
if [ -f "$file" ]; then
echo "File exists."
else
echo "File not found."
fi
Use set -x
to identify why $file
is not found.
Intermediate Debugging
Scenario: Incorrect Variable Assignment
#!/bin/bash
name=JohnDoe # Missing quotes
echo "Hello, $name!"
Debug with bash -x
to see how name
is interpreted.
Advanced Debugging
Scenario: Loop Execution Issue
#!/bin/bash
for i in {1..5}; do
echo "Processing item $i"
done
Run with trap
and DEBUG
to confirm loop logic.
Popular Debugging Tools
ShellCheck
An open-source tool that identifies errors and suggests improvements in your scripts.
sudo apt install shellcheck
shellcheck your_script.sh
strace
A tool for tracing system calls made by your script.
strace -o trace.log bash your_script.sh
grep
Useful for filtering debug logs:
grep "error" debug.log
Use set builtin command
Bash script can tun on or off using set command
- Display commands and their arguments: set -x
- Display shell input lines: set -v
- This may be used to check a shell script for syntax errors. To read commands but do not execute them: set -n
#!/bin/bash
## Turn on debug mode ##
set -x
echo "Users currently on the machine"
w
## Turn OFF debug mode ##
set +x
The screen output terminal:
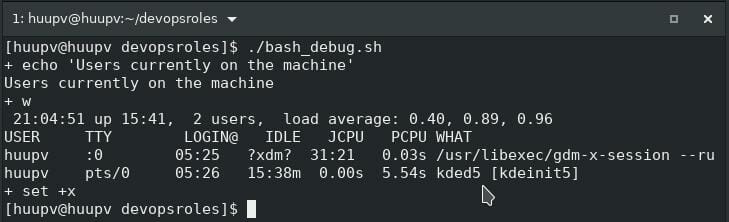
Frequently Asked Questions (FAQ)
1. What is the difference between set -x
and bash -x
?
set -x
: Enables tracing within a script.bash -x
: Traces commands when running a script externally.
2. How do I debug a function in a Bash script?
Use set -x
or echo
within the function to trace its behavior:
my_function() {
set -x
# Function code
set +x
}
3. How can I debug scripts in production?
Redirect debug output to a log file to avoid exposing sensitive information:
bash -x your_script.sh > /var/log/debug.log 2>&1
4. What are the best practices for debugging large scripts?
Break the script into smaller functions or modules. Debug each part individually before integrating.
External Resources
Conclusion
Debugging Bash scripts may seem daunting, but with the right tools and techniques, it becomes manageable. From leveraging set -x
and trap
to employing tools like ShellCheck
and strace
, there’s a method for every scenario. By mastering these strategies, you’ll write more robust and error-free scripts.
Debugging is not just about fixing errors-it’s a learning process that deepens your understanding of Bash. Start with the basics, experiment with advanced tools, and continuously refine your approach. I hope will this your helpful. More details refer to Bash script.