Table of Contents
Introduction
Git is a vital tool for developers and teams in software development, enabling powerful version control for code and project management. Created by Linus Torvalds in 2005, Git offers a distributed version control system where everyone can maintain a complete copy of the project history, enhancing collaboration and reducing risks. In this Git tutorial, we’ll cover the basics, dive into key commands, and look at how to use Git effectively, whether you’re a beginner or an advanced user.
Getting Started with Git
What is Git?
Git is an open-source version control system that tracks changes in files, allowing developers to coordinate work on projects and manage changes over time. Its distributed nature means each user has a full copy of the project history, ensuring better control, collaboration, and faster integration of code.
Why Use Git?
- Version Control: Easily manage code changes and revert back to previous versions.
- Collaboration: Multiple people can work on the same project without conflicts.
- Backup and Recovery: Git serves as a backup for your code and allows you to recover past versions.
- Efficiency: Git makes it easy to test and merge new features or bug fixes without disrupting the main project.
Installing Git
Before starting with Git, you’ll need to install it on your computer.
Steps to Install Git on Different OS
- Windows:
- Download the installer from git-scm.com.
- Run the installer and follow the setup instructions.
- macOS:
- Use Homebrew: Open Terminal and run
brew install git
. - Alternatively, download the Git installer from git-scm.com.
- Use Homebrew: Open Terminal and run
- Linux:
- On Ubuntu/Debian:
sudo apt install git
- On Fedora:
sudo dnf install git
- On Ubuntu/Debian:
To confirm the installation, open a terminal and type:
git --version
Setting Up Git
Once Git is installed, the next step is to configure it.
- Configure Your Identity
Set your username and email, which will be associated with every commit.git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
- Check Configuration Settings
To verify, use:git config --list
- Setting Up a Repository
A repository is a project’s directory managed by Git. You can either create a new repository or clone an existing one.- To create a new repository:
git init
- To clone an existing repository:
git clone [repository-url]
- To create a new repository:
Basic Git Commands
Below are some essential Git commands to help you manage projects effectively.
1. Adding Files to Staging Area
To stage files for commit:
git add [filename]
Or, to add all files:
git add .
2. Committing Changes
Commits are snapshots of your project. Once files are added to the staging area, you can commit them:
git commit -m "Your commit message"
3. Viewing Commit History
To see the history of commits:
git log
4. Branching and Merging
Branches allow you to work on different versions of your project simultaneously.
- Create a New Branch:
git branch [branch-name]
- Switch to a Branch:
git checkout [branch-name]
- Merge Branches: Switch to the main branch and merge your feature branch:
git checkout main git merge [branch-name]
5. Pushing and Pulling Changes
Git works with remote repositories like GitHub or GitLab to allow others to view or contribute.
- Push Changes to Remote:
git push origin [branch-name]
- Pull Changes from Remote:
git pull origin [branch-name]
Advanced Git Commands
1. Resetting Changes
If you need to undo changes, Git provides several reset options:
git reset --soft HEAD~1 # Keeps changes in staging
git reset --hard HEAD~1 # Discards changes completely
2. Reverting Commits
To reverse a commit while retaining history:
git revert [commit-id]
3. Stashing Changes
Stashing lets you save changes for later without committing:
git stash
git stash pop # To retrieve stashed changes
Example Scenarios
Scenario 1: Initializing a New Project
- Create a new project directory and navigate to it.
- Run
git init
to start a repository. - Add files with
git add .
- Commit changes with
git commit -m "Initial commit"
Scenario 2: Collaborating with Team Members
- Clone a shared repository with
git clone [repo-url]
. - Create a new branch for your feature:
git branch feature-branch
. - Make changes, stage, and commit.
- Push your branch to the remote repository:
git push origin feature-branch
.
Git Best Practices
- Commit Often: Regular commits make it easier to track changes.
- Use Descriptive Messages: Write clear and meaningful commit messages.
- Branching Strategy: Use separate branches for new features or fixes.
- Pull Frequently: Regularly pull changes from the remote repository to avoid conflicts.
- Avoid Pushing Broken Code: Ensure your code works before pushing.
FAQ Section
1. What is Git?
Git is a version control system that tracks file changes, allowing developers to manage and collaborate on code efficiently.
2. How is Git different from GitHub?
Git is a tool for version control, while GitHub is a platform that hosts Git repositories, allowing collaboration and code sharing.
3. What is a branch in Git?
A branch is an independent line of development. It allows you to work on different project versions without affecting the main codebase.
4. How do I undo the last commit?
To undo the last commit, use git reset --soft HEAD~1
to keep changes or git reset --hard HEAD~1
to discard them.
5. How can I merge branches in Git?
First, switch to the branch you want to merge into (often main
), then run git merge [branch-name]
.
6. What is Git stash used for?
Git stash temporarily saves changes without committing, allowing you to work on other things without losing progress.
7. How can I view the commit history?
Use git log
to see the commit history, including commit messages, authors, and timestamps.
8. How can I recover deleted files in Git?
If the deletion was committed, use git checkout HEAD~1 -- [filename]
to recover it.
External Resources
For further reading on Git, check out these resources:
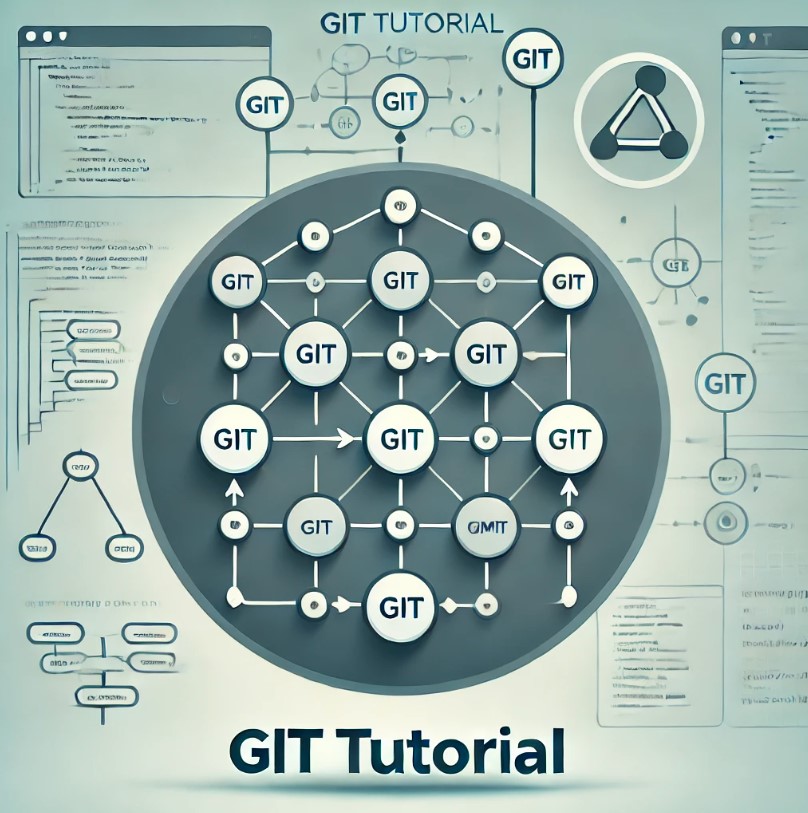
Conclusion
Git is an essential tool for any developer, from hobbyists to professionals. Its version control capabilities offer enhanced collaboration, secure backup, and a streamlined workflow. This Git tutorial covers everything you need to know to get started, from installation to advanced features. Whether you’re working solo or as part of a team, mastering Git will improve your productivity and project management skills. Keep practicing these commands and explore additional resources to deepen your understanding. Thank you for reading the DevopsRoles page!