Table of Contents
Introduction
Jenkins, a widely-used automation server, is a cornerstone of DevOps and Continuous Integration/Continuous Delivery (CI/CD) pipelines. Leveraging Groovy scripting, a dynamic language for the Java platform, empowers users to automate complex tasks, optimize workflows, and extend Jenkins functionalities seamlessly. This guide explores how to utilize Jenkins Automation with Groovy Scripting, providing practical insights and actionable examples.
Why Use Groovy Scripting in Jenkins?
Key Benefits
- Flexibility: Groovy’s dynamic nature simplifies scripting tasks in Jenkins.
- Integration: Seamlessly integrates with Jenkins plugins and Java libraries.
- Efficiency: Automates repetitive tasks, reducing manual intervention.
- Customization: Extends Jenkins’ default capabilities to fit unique project requirements.
Setting Up Jenkins for Groovy Scripting
Prerequisites
- Jenkins Installed: Ensure Jenkins is installed and running.
- Groovy Plugin: Install the Groovy plugin via Jenkins’ Plugin Manager.
- Java Development Kit (JDK): Groovy requires Java to function.
Configuring Jenkins
- Navigate to
Manage Jenkins
>Manage Plugins
. - Search for “Groovy” in the Available Plugins tab.
- Install and restart Jenkins to enable the plugin.
Groovy Scripting Basics
Syntax Overview
Groovy scripts are concise and easy to learn, especially if you’re familiar with Java. Below are basic constructs:
- Variables:
def message = "Hello, Jenkins!"
- Loops:
for (int i = 0; i < 10; i++) { println i }
- Functions:
def greet(name) {
return "Hello, $name!"
}
println greet("Jenkins User")
Automating Jenkins Tasks with Groovy
Example 1: Creating and Configuring a Job
Groovy Script:
import jenkins.model.*
// Create a new job
def jenkins = Jenkins.instance
String jobName = "MyFirstGroovyJob"
def job = jenkins.createProject(hudson.model.FreeStyleProject, jobName)
// Configure job properties
job.description = "This is a job created with Groovy scripting."
job.save()
println "Job $jobName created successfully!"
Example 2: Automating Build Trigger Configurations
Groovy Script:
import hudson.triggers.*
def job = Jenkins.instance.getItem("MyFirstGroovyJob")
job.addTrigger(new SCMTrigger("H/15 * * * *")) // Poll SCM every 15 minutes
job.save()
println "SCM trigger added to job successfully!"
Example 3: Deleting Old Build Artifacts
Groovy Script:
import jenkins.model.*
// Delete build artifacts older than 30 days
Jenkins.instance.getAllItems(Job).each { job ->
job.builds.findAll { it.getTimeInMillis() < System.currentTimeMillis() - (30 * 24 * 60 * 60 * 1000) }.each {
it.delete()
println "Deleted build #${it.number} from ${job.name}"
}
}
Advanced Jenkins Automation with Groovy
Scenario: Dynamic Parameterized Builds
Groovy Script:
import hudson.model.ParametersDefinitionProperty
import hudson.model.StringParameterDefinition
def job = Jenkins.instance.getItem("MyFirstGroovyJob")
def paramsDef = new ParametersDefinitionProperty(
new StringParameterDefinition("ENV", "Development", "Target environment")
)
job.addProperty(paramsDef)
job.save()
println "Added dynamic parameters to job successfully!"
Scenario: Automating Plugin Installations
Groovy Script:
import jenkins.model.Jenkins
import jenkins.plugins.PluginManager
def plugins = ["git", "pipeline"]
def pm = Jenkins.instance.pluginManager
plugins.each { plugin ->
if (!pm.getPlugin(plugin)) {
pm.install(plugin)
println "Installed plugin: $plugin"
} else {
println "Plugin already installed: $plugin"
}
}
FAQ: Jenkins Automation with Groovy Scripting
What is Groovy scripting used for in Jenkins?
Groovy is used to automate tasks, customize jobs, and extend Jenkins’ functionalities beyond its GUI capabilities.
Can I run Groovy scripts directly in Jenkins?
Yes, you can execute scripts using Jenkins’ Script Console (Manage Jenkins > Script Console
).
How do I debug Groovy scripts in Jenkins?
Use println
statements for debugging and check logs under Manage Jenkins > System Log
.
Is Groovy scripting secure?
Always validate and review scripts for security vulnerabilities, especially when handling sensitive data or running on shared servers.
External Resources
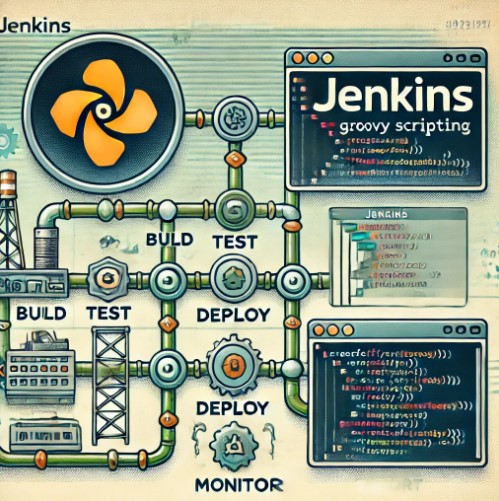
Conclusion
Groovy scripting transforms Jenkins from a robust CI/CD tool into a highly customizable automation powerhouse. Whether creating jobs, managing plugins, or automating workflows, Groovy empowers DevOps professionals to achieve unparalleled efficiency and scalability. Start integrating Groovy into your Jenkins pipelines today to unlock its full potential. Thank you for reading the DevopsRoles page!