Table of Contents
Introduction
Docker has revolutionized the way we develop, deploy, and manage applications by enabling lightweight, portable containers. However, without proper optimization, Docker containers can consume excessive resources, degrade performance, and increase operational costs. In this comprehensive guide, we’ll explore strategies, tips, and practical examples to achieve effective Docker optimization.
Why Docker Optimization Matters
Optimizing Docker containers is crucial for:
- Enhanced Performance: Reduced latency and improved response times.
- Lower Resource Usage: Efficient utilization of CPU, memory, and storage.
- Cost Savings: Minimized infrastructure expenses.
- Scalability: Seamless scaling of applications to meet demand.
- Stability: Prevention of resource contention and crashes.
Let’s dive into practical methods to optimize Docker containers.
Key Strategies for Docker Optimization
1. Optimize Docker Images
Docker images are the building blocks of containers. Reducing their size can significantly improve performance.
Techniques to Optimize Docker Images:
Use Minimal Base Images: Choose lightweight base images like alpine
instead of ubuntu
.
FROM alpine:latest
Multi-Stage Builds: Separate build and runtime stages to eliminate unnecessary files.
# Stage 1: Build
FROM golang:1.18 AS builder
WORKDIR /app
COPY . .
RUN go build -o main .
# Stage 2: Runtime
FROM alpine:latest
WORKDIR /app
COPY --from=builder /app/main .
CMD ["./main"]
Clean Up Temporary Files: Remove unused files and dependencies during image creation.
RUN apt-get update && apt-get install -y curl && rm -rf /var/lib/apt/lists/*
2. Efficient Container Management
Managing containers effectively ensures optimal resource allocation.
Best Practices:
- Limit Resources: Set resource limits to prevent containers from monopolizing CPU or memory.
docker run --memory="512m" --cpus="1.5" my-container
- Remove Unused Containers: Regularly clean up stopped containers and unused images.
docker system prune -a
- Use Shared Volumes: Avoid duplicating data by leveraging Docker volumes.
docker run -v /data:/app/data my-container
3. Optimize Networking
Efficient networking ensures faster communication between containers and external services.
Tips:
- Use Bridge Networks: For isolated container groups.
- Enable Host Networking: For containers requiring minimal latency.
docker run --network host my-container
- Reduce DNS Lookups: Cache DNS results within containers to improve resolution times.
4. Monitor and Analyze Performance
Monitoring tools help identify bottlenecks and optimize container performance.
Recommended Tools:
- Docker Stats: In-built command to monitor resource usage.
docker stats
- cAdvisor: Detailed container metrics visualization.
docker run -d --volume=/:/rootfs:ro --volume=/var/run:/var/run:rw --volume=/sys:/sys:ro --volume=/var/lib/docker/:/var/lib/docker:ro --publish=8080:8080 google/cadvisor
- Prometheus and Grafana: Advanced monitoring and dashboarding solutions.
5. Automate Optimization
Automating repetitive tasks improves consistency and reduces manual errors.
Examples:
- Use Docker Compose: Automate multi-container deployments.
version: '3.8'
services:
web:
image: nginx:latest
ports:
- "80:80"
app:
image: my-app:latest
depends_on:
- web
- CI/CD Integration: Use pipelines to automate image building, testing, and deployment.
Examples of Docker Optimization in Action
Example 1: Reducing Image Size
Before Optimization:
FROM ubuntu:latest
RUN apt-get update && apt-get install -y python3
COPY . /app
CMD ["python3", "app.py"]
After Optimization:
FROM python:3.9-slim
COPY . /app
CMD ["python", "app.py"]
Example 2: Limiting Resources
Command:
docker run --memory="256m" --cpus="1" optimized-container
FAQ: Docker Optimization
1. What is Docker optimization?
Docker optimization involves improving container performance, reducing resource usage, and enhancing scalability through best practices and tools.
2. How can I reduce Docker image size?
Use minimal base images, multi-stage builds, and clean up unnecessary files during the build process.
3. What tools are available for monitoring Docker performance?
Popular tools include Docker Stats, cAdvisor, Prometheus, and Grafana.
4. Why set resource limits for containers?
Setting resource limits prevents a single container from overusing resources, ensuring stability for other applications.
5. Can automation improve Docker optimization?
Yes, automating tasks like image building, testing, and deployment ensures consistency and saves time.
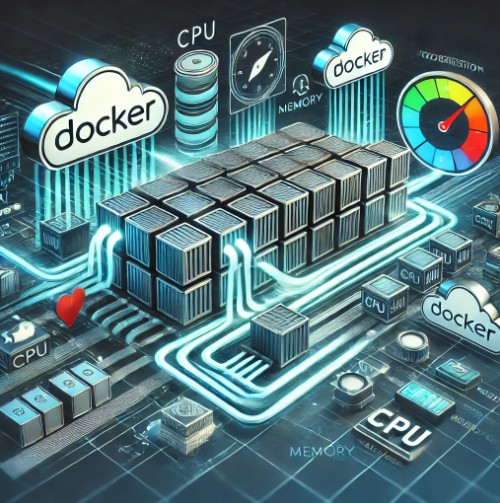
External Resources
Conclusion
Docker optimization is essential for ensuring efficient, cost-effective, and scalable containerized applications. By applying the strategies outlined in this guide—from optimizing images and managing containers to monitoring performance and automating processes—you can unlock the full potential of Docker in your development and production environments.
Start optimizing your Docker containers today and experience the difference in performance and efficiency. Thank you for reading the DevopsRoles page!