Table of Contents
Introduction
Logs are the backbone of system diagnostics, security auditing, and application performance monitoring. Efficient log management is crucial for identifying issues and maintaining optimal system performance. Bash scripting provides a powerful and versatile toolset for automating log management tasks, from parsing logs to archiving and alerting. In this article, we will explore how to use Bash scripting to streamline log management processes effectively.
Why Use Bash Scripting for Log Management?
Bash scripting offers simplicity, flexibility, and robust capabilities to handle various log management tasks. Here are some reasons why it’s an excellent choice:
- Automation: Automate repetitive tasks like log rotation, compression, and deletion.
- Efficiency: Process large log files quickly using command-line utilities.
- Customizability: Tailor scripts to specific log formats and requirements.
- Integration: Seamlessly integrate with other tools and workflows in Unix/Linux environments.
Key Bash Commands for Log Management
tail
The tail
command displays the last few lines of a log file, useful for monitoring real-time updates.
# Monitor a log file in real-time
$ tail -f /var/log/syslog
grep
Search for specific patterns within log files to filter relevant information.
# Find error messages in a log file
$ grep "ERROR" /var/log/syslog
awk
Extract and process structured log data.
# Extract IP addresses from logs
$ awk '{print $1}' /var/log/access.log
sed
Edit log files in-place to modify content programmatically.
# Remove sensitive information from logs
$ sed -i 's/password=.*$/password=****/' /var/log/app.log
find
Locate and manage old log files.
# Delete log files older than 7 days
$ find /var/log -type f -mtime +7 -exec rm {} \;
Bash Scripting for Common Log Management Tasks
Log Rotation
Automating log rotation ensures that logs donβt consume excessive disk space.
#!/bin/bash
# Rotate logs
LOG_DIR="/var/log/myapp"
ARCHIVE_DIR="/var/log/myapp/archive"
mkdir -p "$ARCHIVE_DIR"
for LOG_FILE in $LOG_DIR/*.log; do
TIMESTAMP=$(date +"%Y%m%d_%H%M%S")
mv "$LOG_FILE" "$ARCHIVE_DIR/$(basename "$LOG_FILE" .log)_$TIMESTAMP.log"
gzip "$ARCHIVE_DIR/$(basename "$LOG_FILE" .log)_$TIMESTAMP.log"
touch "$LOG_FILE"
done
Log Parsing
Parsing logs for specific events or errors can aid troubleshooting.
#!/bin/bash
# Parse logs for errors
LOG_FILE="/var/log/syslog"
ERROR_LOG="/var/log/error_report.log"
grep "ERROR" "$LOG_FILE" > "$ERROR_LOG"
echo "Errors extracted to $ERROR_LOG"
Archiving and Cleanup
Archive old logs and free up disk space by removing stale files.
#!/bin/bash
# Archive and clean up old logs
LOG_DIR="/var/log/myapp"
ARCHIVE_DIR="/var/log/myapp/archive"
mkdir -p "$ARCHIVE_DIR"
find "$LOG_DIR" -type f -mtime +30 -exec gzip {} \; -exec mv {} "$ARCHIVE_DIR" \;
Real-Time Alerts
Send notifications when critical events occur.
#!/bin/bash
# Monitor logs and send alerts
LOG_FILE="/var/log/syslog"
PATTERN="CRITICAL"
ALERT_EMAIL="admin@example.com"
tail -f "$LOG_FILE" | while read LINE; do
echo "$LINE" | grep -q "$PATTERN"
if [ $? -eq 0 ]; then
echo "$LINE" | mail -s "Critical Alert" "$ALERT_EMAIL"
fi
done
Advanced Scenarios
Centralized Log Management
Combine Bash with tools like rsync
to centralize logs from multiple servers.
#!/bin/bash
# Collect logs from remote servers
REMOTE_SERVERS=("server1" "server2")
DEST_DIR="/var/log/central"
mkdir -p "$DEST_DIR"
for SERVER in "${REMOTE_SERVERS[@]}"; do
rsync -avz "user@$SERVER:/var/log/*" "$DEST_DIR/$SERVER/"
done
Analyzing Logs with Visualization Tools
Export logs to formats compatible with visualization tools like ELK stack.
#!/bin/bash
# Convert logs to JSON format
LOG_FILE="/var/log/access.log"
JSON_FILE="/var/log/access.json"
awk '{print "{\"ip\":\"" $1 "\",\"timestamp\":\"" $4 "\"}"}' "$LOG_FILE" > "$JSON_FILE"
FAQs
What are the benefits of automating log management with Bash?
Automation reduces manual effort, minimizes errors, and ensures timely log processing.
Can Bash handle very large log files?
Yes, but for extremely large files, consider tools like logrotate
or distributed solutions.
How do I secure sensitive data in logs?
Use commands like sed
to redact sensitive information before storage or sharing.
What tools complement Bash scripting for log management?
Tools like logrotate
, ELK stack, and Splunk integrate well with Bash for enhanced capabilities.
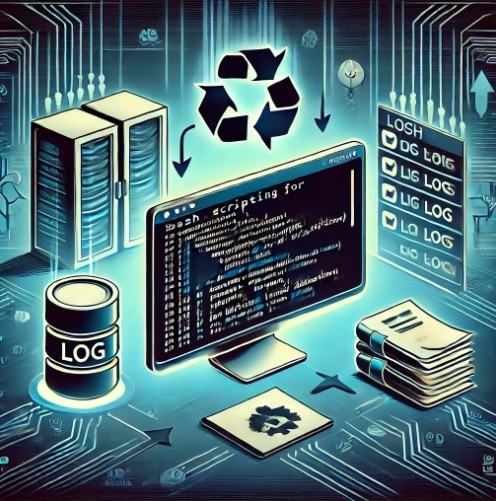
External Links
Conclusion
Bash scripting is an indispensable tool for efficient log management. By automating tasks like log rotation, parsing, and alerting, you can save time and ensure your systems run smoothly. Start implementing these techniques today and streamline your log management workflow! Thank you for reading theΒ DevopsRolesΒ page!