Table of Contents
Introduction
Python is a versatile and powerful programming language widely used for various applications, from web development to data analysis. One of the fundamental skills in Python is string manipulation, particularly concatenating and splitting strings. This article will guide you through the basics and more advanced techniques for concatenate and Split Strings in Python
, complete with practical examples and frequently asked questions.
What is String Concatenation?
String concatenation is the process of joining two or more strings end-to-end to form a single string. This operation is common in programming when constructing messages, creating paths, or merging data.
Basic String Concatenation in Python
In Python, there are several ways to concatenate strings. Let’s start with the most basic methods:
Using the +
Operator
The +
operator is the simplest way to concatenate strings in Python.
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # Output: Hello World
Using the join()
Method
The join()
method is useful when you need to concatenate multiple strings from a list or tuple.
words = ["Hello", "World"]
result = " ".join(words)
print(result) # Output: Hello World
Using Formatted String Literals (f-strings)
Introduced in Python 3.6, f-strings provide a readable and concise way to concatenate strings.
name = "Alice"
greeting = f"Hello, {name}!"
print(greeting) # Output: Hello, Alice!
Advanced String Concatenation Techniques
Using the %
Operator
The %
operator, also known as the string formatting operator, is an older method for concatenating strings.
name = "Bob"
age = 25
result = "My name is %s and I am %d years old." % (name, age)
print(result) # Output: My name is Bob and I am 25 years old.
Using the format()
Method
The format()
method allows for more complex string formatting and concatenation.
name = "Carol"
age = 30
result = "My name is {} and I am {} years old.".format(name, age)
print(result) # Output: My name is Carol and I am 30 years old.
What is String Splitting?
String splitting is the process of breaking down a string into a list of substrings based on a delimiter. This is particularly useful when parsing data from files, user input, or network responses.
Basic String Splitting in Python
Using the split()
Method
The split()
method is the most common way to split strings in Python.
sentence = "Hello World"
words = sentence.split()
print(words) # Output: ['Hello', 'World']
Splitting with a Specific Delimiter
You can specify a delimiter to split the string by passing it as an argument to the split()
method.
data = "apple,banana,cherry"
fruits = data.split(',')
print(fruits) # Output: ['apple', 'banana', 'cherry']
Advanced String Splitting Techniques
Using Regular Expressions
For more complex splitting patterns, you can use the re
module.
import re
text = "one1two2three3"
result = re.split(r'\d', text)
print(result) # Output: ['one', 'two', 'three', '']
Limiting Splits
You can limit the number of splits by passing a second argument to the split()
method.
data = "apple,banana,cherry,dates"
fruits = data.split(',', 2)
print(fruits) # Output: ['apple', 'banana', 'cherry,dates']
Practical Example
In this tutorial, we will demonstrate how to split and concatenate a string in Python using a practical example.
Example
Given an initial string Dev,Ops,R,o,l.e.s.com
, we will split it and then concatenate the parts to form a new string "DevOpsRolescom"
.
string = "Dev,Ops,R,o,l.e.s.com"
print("Initial Strings: {}".format(string))
cleanstring = ''
for i in range(len(string)):
if string[i].isalpha():
cleanstring += string[i]
newcleanstring = str(cleanstring)
print("New Strings: {}".format(newcleanstring))
Output:
Initial Strings: Dev,Ops,R,o,l.e.s.com
New Strings: DevOpsRolescom
This example shows how to filter out only the alphabetic characters from the initial string and concatenate them to form a clean string.
The result is the picture below:
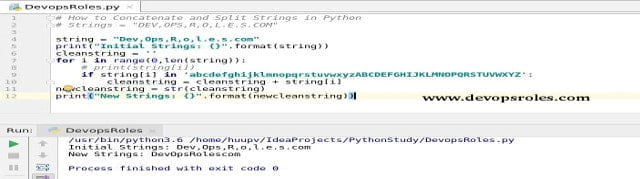
Common Issues and Solutions
Issue: Leading and Trailing Spaces
When concatenating strings, you may encounter unwanted spaces. Use the strip()
method to remove them.
str1 = " Hello "
str2 = " World "
result = (str1 + str2).strip()
print(result) # Output: Hello World
Issue: Handling None Values
When concatenating strings that might include None
values, use conditional expressions or the or
operator.
str1 = "Hello"
str2 = None
result = str1 + (str2 or "")
print(result) # Output: Hello
Frequently Asked Questions
How do you concatenate multiple strings efficiently?
For concatenating a large number of strings, use the join()
method with a list of strings for better performance.
Can you concatenate strings with different data types?
Yes, but you need to convert non-string data types to strings using the str()
function.
num = 100
result = "The number is " + str(num)
print(result) # Output: The number is 100
How do you split a string into characters?
Use the list()
function to split a string into a list of characters.
word = "hello"
characters = list(word)
print(characters) # Output: ['h', 'e', 'l', 'l', 'o']
Conclusion
Concatenating and splitting strings are fundamental skills in Python programming. This guide has covered both basic and advanced techniques to help you manipulate strings efficiently. Whether you are building a simple script or a complex application, mastering these techniques will enhance your coding capabilities.
By understanding and utilizing the methods and examples provided, you’ll be able to handle various string manipulation tasks in Python with confidence. Keep practicing, and soon these techniques will become second nature.
Thank you for reading the DevopsRoles page!